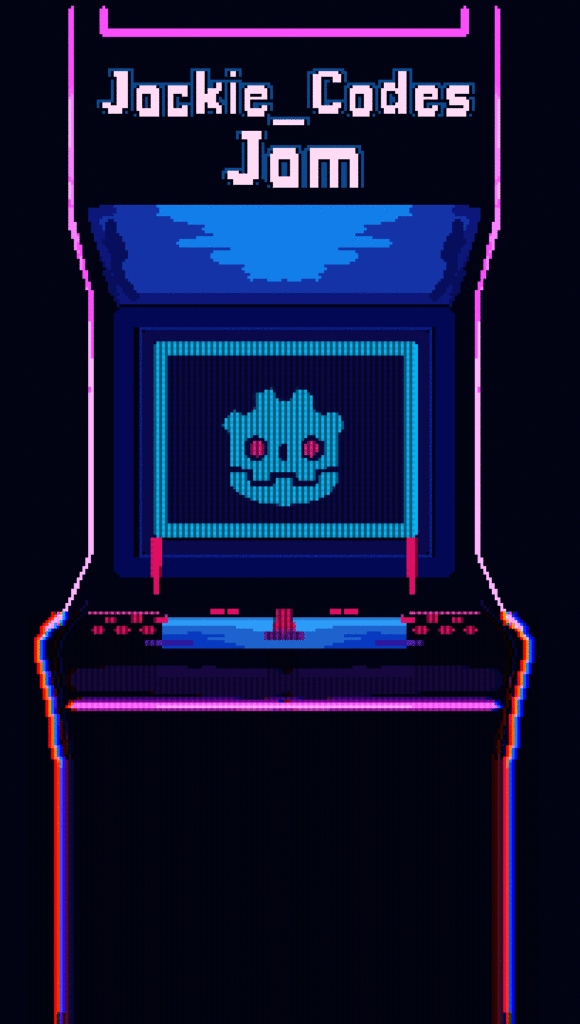
This was the first game jam I had ever participated in. It was also the first game I ever wrote. That being said, While I did learn a lot, I feel like there isn’t going to be quite as much to unpack and debrief for my first jam. 100% of what I accomplished was newly learnt. I spent the entire jam just planning on getting something done and, as long as I accomplished that, I would have felt good about my first jam. That isn’t to say that I had no notes or important takeaways. What it does mean is that it’s difficult to pick out discrete things that I learned that I think might be widely applicable to other people.
It’s okay to not have a plan
But I wouldn’t recommend it. I’m not going to lie, I think I just got extremely lucky and was also able to leverage my background of creative solutioning to come up with clever answers to difficult questions that I caused myself to deal with. Having said that, jumping in headfirst without a clear, concise plan and figuring it out as you go is totally an option. The success of this strategy may be partially due to just how my brain is wired, ADHD and all. With Thor always beating the GDD drum and stressing how important a plan is, I was prepared for disaster.
This isn’t to say you shouldn’t have a plan. Heck, I’m not even discouraging preplanning or brainstorming, writing an outline, or even a GDD. The point I want to emphasize is to not get paralyzed by indecision if you’re not instantly inspired once a jam’s theme is revealed. Sometimes you just need to poke around asset stores online (even if you end up making the assets yourself) or even play around in the engine with new mechanics or designs to see if you can spark any inspiration.
This was all about 3 months ago (at the time of me writing this) so my memory is a bit fuzzy, but I think my approach was as follows. I first Googled about alchemy, despite having a cursory knowledge of what it is, I wanted to see if anything about its wiki page or historical usage might spark any sort of inspiration. I know most common perceptions of “alchemy” focus around making potions, but as defined by Google, alchemy is:
the medieval forerunner of chemistry, based on the supposed transformation of matter. It was concerned particularly with attempts to convert base metals into gold or to find a universal elixir.
“Transformation of matter” from this definition really grabbed at my brain. And from there, the rest of the game really flowed, almost stream of consciousness style.
It’s okay to refactor during a jam
I by no means recommend major refactors, but if you have the time and it’s to learn a new design pattern, learn a new technique, or challenge yourself in some way, I consider that a noble enough cause to risk it. In particular, I redesigned the character controller inside Lazlo’s pretty much from the ground-up halfway through the game jam. Lacking any experience at all with Godot, I just dove in the deep end and started coding game mechanics, throwing 70% of my player code inside the _process_physics(delta)
function. While this isn’t the end of the world, the resulting spaghetti code really suffocated my ability to quickly and painlessly implement or tweak mechanics without untangling the state logic and writing a dozen if
statements to make sure I wasn’t running logic at the wrong point in time.
The irony isn’t lost on me that, had I planned ahead, I wouldn’t have backed myself into a corner, struggling to make sense of all the twisted, intertwined, interdependent state logic strewn about my player script. But to me, game jams should be primarily about learning. That’s always my primary goal, at least. Mistakes are the most potent form of education. If you did everything perfectly the first time, then you come out on the other side of the experience, unchanged. Sometimes you just have to learn the hard way. In writing convoluted, interdependent, messy code, I experienced, firsthand, the difference and value brought to the table by implementing a finite state machine.
Finite state machines are all the rage
They really do make organizing your code way easier. They also help you sort your thoughts out and compartmentalize code so there aren’t side effects and code processes only when it should. Not much more to say on this topic, I might post about state machines cause they’re such an in-depth topic, but pretty much you should implement a finite state machine in your game if you’re struggling to untangle character logic. I followed a pretty simple tutorial about state machines, but the trickiest part of it all was adding this in closer to the end of the jam. I had to take my largely complex and incredibly messy player script and essentially gut it and split it up into basic states. Going forward, I’ll make sure I implement a finite state machine from the very beginning, where applicable, to avoid this insane headache. Despite being possibly the most stressful part of my project, converting to using a state machine was ultimately worth it as it decoupled all the state logic from the player script, organizing and modularizing it into separate scripts, one for each state.
Colors matter
The most prevalent feedback I got from the jam was that I needed to be more cognizant about the color palette and how I was using it than I had been. Being the one who designed the whole game, I was blind to the poor art decisions I had made, primarily regarding decorative support pillars. I can’t tell you what behooved me to make the brighter, higher-contrast pillar represent the non-collidable pillars in the background, and the darker, lower contrast pillars represent foreground, collidable pillars. All I can tell you is that I don’t think I gave it much thought. Almost universally, though, the main feedback I got was in regards to how confusing that art decision was.
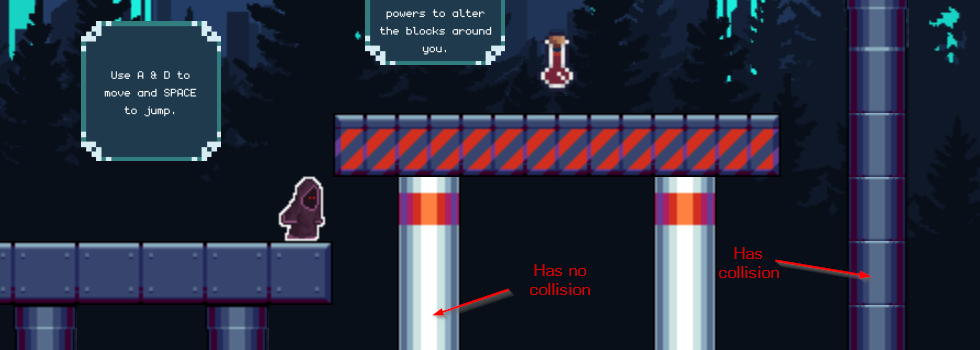
Call down, signal up
If you frequent the Godot forums, the subreddit, or the Discord (invite can be found on their community page), you might see this phrase repeated: “call down, signal up“. And, frankly, it’s parroted like a broken record for a good reason. It encourages good and reliable practices, avoids convoluted relationships that are prone to breaking, and helps to structure a project in an expected manner. This pattern describes the methods in which you should access objects and properties. If you’re traversing down the node tree, you can reference the children nodes to call functions and read or modify properties. If you’re traversing up the node tree, you need to have the child node emit a signal that the parent will connect to in order to achieve the outcome you want. If you know what to expect, given a certain tree structure, about how nodes are to interact, then feature implementation and troubleshooting occur more naturally and smoothly. That being said…
@export node references
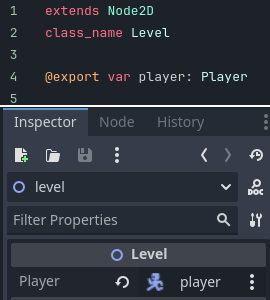
Within a self-contained scene, complex tree traversal can be solved simply by exporting a variable and linking the target node in the inspector. This was particularly useful in the state machine implementation, where I needed different states to reference each other. Furthermore, if you strictly type your exports, and your nodes have class_name
defined, you can make the editor make sure you grab the correct node (or at least the correct type of node) and enable editor hints when you’re using the node. This was especially useful when it came to the state machine states each time a state needed to reference another state.